Ricardo Teixeira wrote:Looking forward to any additional information, this is actually something that we would love to improve in AT2, it could affect a few other uses.
Textures are changed every time the virtual texture is updated or rebuilt, the automated AT2 scene conversion tools also resizes them.
So, getting back to topic, unfortunately, a way 2 didn't work as expected, shaders do not fall back to non-AT ones, so currently we are stuck at what we have now.
The code that generates the cubemap looks like this:
- Code: Select all
public class RealtimeReflection : MonoBehavior
{
public Camera TargetProbe;
private RenderTexture _reflectionCubemap;
private bool _updateQueued = false;
private Coroutine _current;
public void Start()
{
_reflectionCubemap = new RenderTexture(512, 512, 0);
_reflectionCubemap.dimension = TextureDimension.Cube;
UpdateReflection();
}
public void UpdateReflection()
{
if (_updateQueued)
{
StopCoroutine(_current);
_updateQueued = false;
}
_current = StartCoroutine(UpdateReflectionImpl());
}
private float delay = 0.5f;
private IEnumerator UpdateReflectionImpl()
{
TargetProbe.enabled = true;
TargetProbe.transform.rotation = Quaternion.Euler(0, 0, 0);
yield return new WaitForEndOfFrame();
TargetProbe.RenderToCubemap(_reflectionCubemap, 1);
yield return new WaitForEndOfFrame();
TargetProbe.transform.rotation = Quaternion.Euler(0, -90, 0);
yield return new WaitForEndOfFrame();
TargetProbe.RenderToCubemap(_reflectionCubemap, 2);
yield return new WaitForEndOfFrame();
TargetProbe.transform.rotation = Quaternion.Euler(-90, 0, 0);
yield return new WaitForEndOfFrame();
TargetProbe.RenderToCubemap(_reflectionCubemap, 4);
yield return new WaitForEndOfFrame();
TargetProbe.transform.rotation = Quaternion.Euler(90, 0, 0);
yield return new WaitForEndOfFrame();
TargetProbe.RenderToCubemap(_reflectionCubemap, 8);
yield return new WaitForEndOfFrame();
TargetProbe.transform.rotation = Quaternion.Euler(0, -180, 0);
yield return new WaitForEndOfFrame();
TargetProbe.RenderToCubemap(_reflectionCubemap, 32);
yield return new WaitForEndOfFrame();
TargetProbe.enabled = false;
_updateQueued = true;
}
public void Update()
{
if (_updateQueued)
{
this.GetComponent<MeshRenderer>().material.SetTexture("_Cube", _reflectionCubemap);
_updateQueued = false;
}
}
One side of the cubemap is not not rendered, because it's not visible. This is intentional. Small optimization.
The result looks like this:
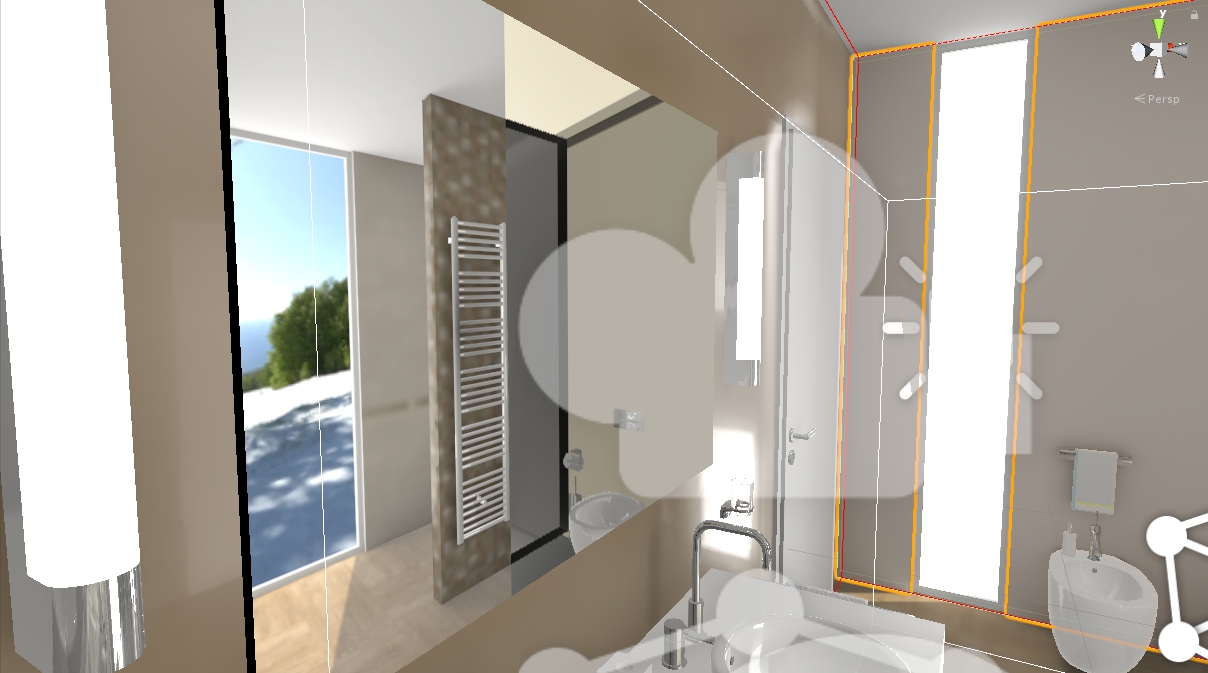
You can see that the side that contains a toilet and bidet has it's geometry flipped, textures are blurred.
This is what camera looks like:
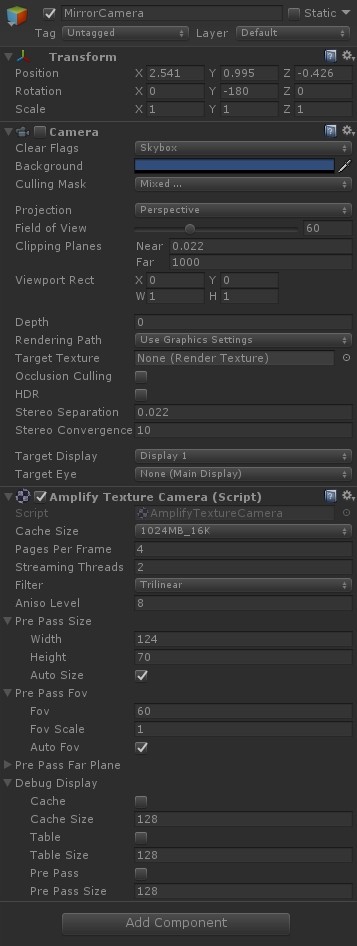
If I disable Amplify Texture Camera component the result looks like this:
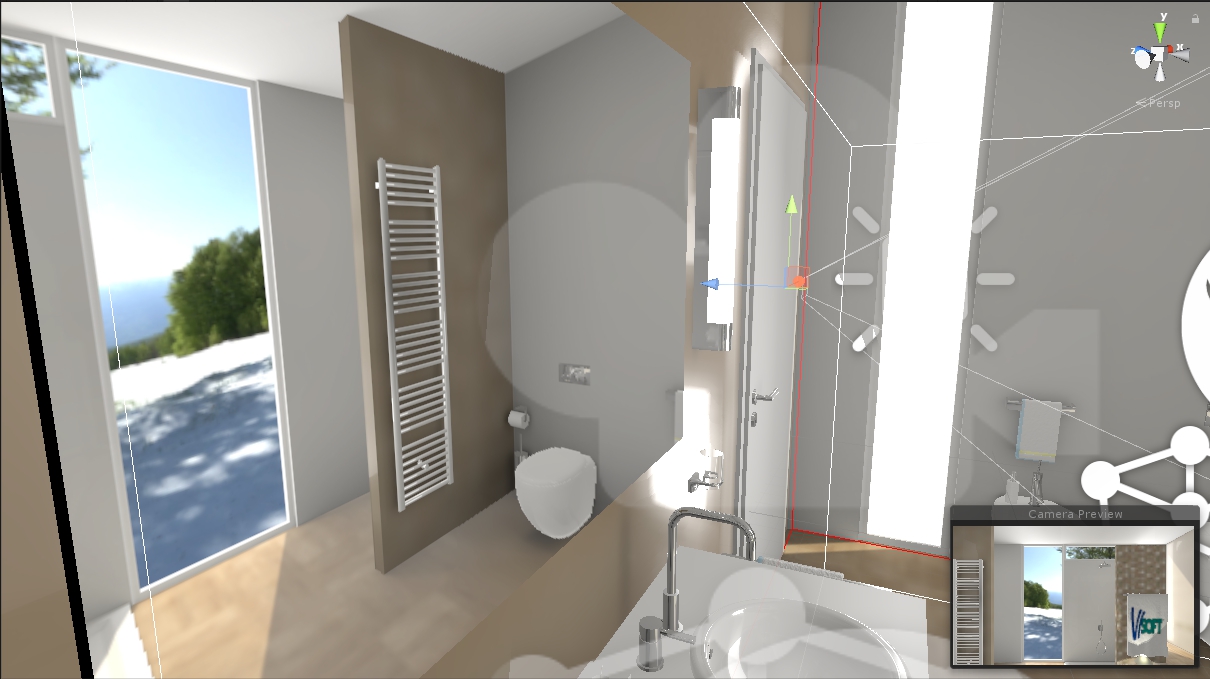
As you can see, geometry flipping is gone and camera renders fine, except for blurred textures, obviously.
Some kind of workaround for this issue would be really nice. We are using Unity 5.5.2 and AT2 trial.